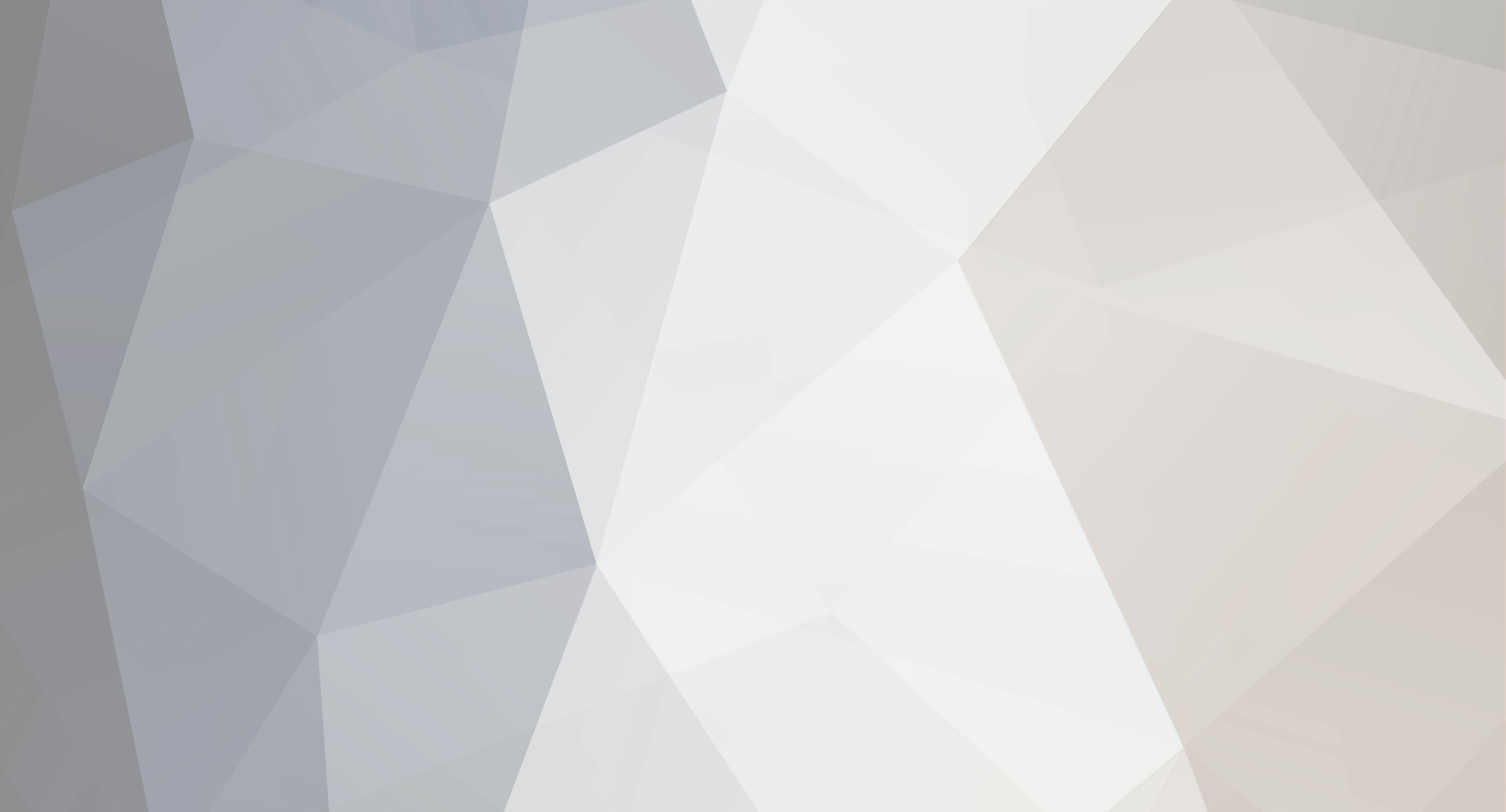

MrPineapple
Member-
Content Count
19 -
Joined
-
Last visited
-
Medals
-
Medals
-
Community Reputation
11 GoodAbout MrPineapple
-
Rank
Private First Class
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
garrison units script - random place units in buildings
MrPineapple replied to MrPineapple's topic in ARMA 3 - MISSION EDITING & SCRIPTING
updated to use Params command for input validation -
garrison units script - random place units in buildings
MrPineapple posted a topic in ARMA 3 - MISSION EDITING & SCRIPTING
Here is a simple script that spawns units in buildings randomly. I got tired of hand placing units with the 3d editor. // populates building positions with units according to parameters /* 0: center position to search for houses (position) 1: radius to search for (number) 2: spawn chance to spawn unit in house position (0.0-1.0) 3: max number of units to spawn (number) 4: array of classnames to spawn (array of strings) 5: side of units to spawn (side) 6: OPTIONAL: DisableAI "MOVE" (bool) // stops ai from moving 7: OPTIONAL: SetUnitPos "UP" (bool) // keeps unit standing */ Private ["_basePos", "_radius", "_spawnChance", "_maxUnits", "_aryManClass", "_side", "_disableMove", "_enableStanding"]; Private ["_spawnedUnits", "_aryHouse", "_aryHousePos", "_aryUnits", "_grp"]; Params [["_basePos",[0,0,0],[[]],3], ["_radius",100,[0]], ["_spawnChance",0.5,[0.0]], ["_maxUnits",10,[0]], ["_aryManClass",["I_Soldier_F"],[[]]], ["_side",resistance,[west]], ["_disableMove",false,[false]], ["_enableStanding",false,[false]]]; _spawnedUnits = 0; // holds total number of spawned units // get array of nearby houses _aryHouse = []; _aryHouse = NearestObjects [_basePos, ["House"], _radius]; _aryHousePos = []; // get array of house positions for all houses { Private ["_house", "_ary"]; _house = _x; _ary = [_x] Call BIS_fnc_BuildingPositions; if (Count _ary > 0) then { _aryHousePos = _aryHousePos + _ary; }; } foreach _aryHouse; // shuffle house positions _aryHousePos = _aryHousePos Call BIS_fnc_ArrayShuffle; _aryUnits = []; // create random units for each of the remaining house positions _grp = CreateGroup _side; _grp EnableAttack false; // leader won't issue attack commands { Private ["_pos", "_manClass", "_man"]; _pos = _x; if ( ((Random 100)/100) < _spawnChance ) then { if (_spawnedUnits < _maxUnits) then { _spawnedUnits = _spawnedUnits + 1; _manClass = _aryManClass Call BIS_fnc_SelectRandom; _man = _grp CreateUnit [_manClass, _pos, [], 0, "NONE"]; _man SetPos _pos; _man SetDir (Random 360); DoStop _man; if (_disableMove) then {_man DisableAI "MOVE"}; if (_enableStanding) then {_man SetUnitPos "UP"}; _aryUnits Set [(Count _aryUnits), _man]; //SystemChat str(_spawnedUnits); }; }; // terminate extra iterations if max units reached if (_spawnedUnits >= _maxUnits) Exitwith {}; } foreach _aryHousePos; _aryUnits here is how to execute the script: assuming you named the script "garrison_units.sqf" Parameters: 0: center position to search for houses (position) 1: radius to search for (number) 2: spawn chance to spawn unit in house position (0.0-1.0) 3: max number of units to spawn (number) 4: array of classnames to spawn (array of strings) 5: side of units to spawn (side) 6: OPTIONAL: DisableAI "MOVE" (bool) // stops ai from moving 7: OPTIONAL: SetUnitPos "UP" (bool) // keeps unit standing Returns: array of spawned units if (IsServer) then { _units = [GetPos Player, 100, 0.5, 50, ["I_Soldier_F","I_Soldier_AR_F","I_Soldier_GL_F"], resistance] Call Compile PreprocessFileLineNumbers "garrison_units.sqf"; { _x SetSkill["AimingAccuracy",0.0]; } foreach _units; }; I believe you should run the script server side only -
Clients spawn as birds in MP
MrPineapple replied to MrPineapple's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Thank you for the help. I think I'll just stick to singleplayer. I tried making my mission multiplayer compatible. It didn't turn out the way I thought. -
Clients spawn as birds in MP
MrPineapple replied to MrPineapple's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I tried that but it still doesn't work. At least it works in singleplayer. It would just be nice to play with other people. -
Clients spawn as birds in MP
MrPineapple replied to MrPineapple's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I tried removing the sunday_revive but my friend keeps spawning as a bird. I don't know why. Does this code in the description.ext have anything to do with it? RespawnTemplates[] = {"Group", "Spectator"}; -
Hello, I can't figure out why clients spawn as birds. I as the host with AI spawn just fine. Could someone please help me figure out why clients aren't spawning like they should. Here is the mission, I would be grateful for any help. mission
-
RscCombo + lbsetpicture = black color of the picture!
MrPineapple replied to jts_2009's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Add this to your combobox: colorPicture[] = {1,1,1,1}; colorPictureSelected[] = {1,1,1,1}; colorPictureDisabled[] = {1,1,1,1}; colorPictudeDisabled[] = {1,1,1,0.25}; I had a problem with black pictures and adding those lines fixed it. The picture color in listboxes/comboboxes seems to have been recently added. -
Using functions inside a script?
MrPineapple replied to jens198's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Those functions should be local variables not global variables if they are only used in that script. You have, which are global variables: setupLeader = {}; setupRifleman = {}; setupMachingunner = {}; You can use instead; these functions will only be local to this script: _setupLeader = {}; _setupRifleman = {}; _setupMachingunner = {}; So put an underscore in front of those function variables if they are only needed in that one script. edit: nevermind I didn't see that you actually do want the functions global because your using 'bis_fnc_mp'. You said in the title functions inside scripts. So I interpreted it as you wanting local functions. -
How to kill certain # of hostiles and end task
MrPineapple replied to jolteon00's topic in ARMA 3 - MISSION EDITING & SCRIPTING
((UNITNAME [url="https://community.bistudio.com/wiki/countEnemy"]countEnemy[/url] thislist) <= 5) && (({side _x == civilian && damage _x <= 0.25} count thislist) == ({side _x == civilian} count thislist)) Name your player unit and use that in place of UNITNAME. Only 5 or less units in the trigger area can be enemy to specified unit. All units in trigger area that are civilian must have health greater/equal to 75%. The trigger activation would be set to 'ANYBODY'. -
Use forceWeatherChange for immediate overcast transition.
-
Event Handler for the spawning of units / groups / vehicles etc...
MrPineapple replied to jaynic's topic in ARMA 3 - MISSION EDITING & SCRIPTING
You can use the Zeus eventhandler CuratorGroupPlaced. It fires when a new group is placed by Zeus. Use units command to get array of group's units. Then in the eventhandler execute your script passing the array of units for that group to it. In your script use foreach command to loop through your units. Use a switch statement to equip your units based on their classname with the 'default' block as your simple infantry loadout for unspecified classnames. Maybe just executing a loadout script for that unit. _group = _this; //spawned group passed to script { //determine side of unit switch (side _x) do { case west: { //determine classname of unit switch (toLower(typeOf _x)) do { default { _handle = [_x] execVM 'loadout.sqf'; waitUntil{scriptDone _handle}; }; //unspecified classname case "b_soldier_at": { _handle = [_x] execVM 'loadout.sqf'; waitUntil{scriptDone _handle}; }; case "b_soldier_aa": { _handle = [_x] execVM 'loadout.sqf'; waitUntil{scriptDone _handle}; }; }; }; case east: { }; case resistance: { }; default //civilian { }; }; } forEach (units _group); -
Arma 3 modules: Easy editing for noobs
MrPineapple replied to thelegendarykhan's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Use the "-window" and "-nopause" commandline parameters when launching the game. "-window" starts game in window mode, or press "alt + enter" during game to switch between window/fullscreen "-nopause" keeps the game running even if the window isn't currently active, such as looking in your mission folder. "alt + tab" for cycling windows and "alt + shift + tab" for cycling windows in reverse direction. -
SAMO Squad Action Menu Orders
MrPineapple replied to mikey74's topic in ARMA 3 - ADDONS & MODS: COMPLETE
You could make a custom menu and use showCommandingMenu accessible with a key press if you don't want to put the actions in the scroll menu. Or a key press and one action in the scroll menu to access the custom menu. I think if you define the custom menu in the config, then you can replicate some of the commands from the command menu. -
CH View Distance Script
MrPineapple replied to champ-1's topic in ARMA 3 - MISSION EDITING & SCRIPTING
A simple suggestion would be to use a combo box for the grass setting instead with the same options as the terrain setting in the graphics options. You could have a selection changed event for the combo box to set the grass setting with the value you set with lbSetValue Here are the grass values based on the terrain setting in the graphics options. TerrainGrid values: Low = 50 (NoGrass) Standard = 25 High = 12.5 Very High = 6.25 Ultra = 3.125 -
arma 3 multiplayer has got out of control for new people
MrPineapple replied to Tyl3r99's topic in ARMA 3 - GENERAL
Here is a server browser that I find so much better than the vanilla browser. Server Browser It is an external application that launches you directly into the server you choose, supports multiple profiles with different mod setups, is more convenient, and loads all the servers faster.