Sign in to follow this
Followers
0
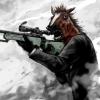
Arma 2 - My time management script
By
wyattwic, in ARMA 2 & OA : MISSIONS - Editing & Scripting
By
wyattwic, in ARMA 2 & OA : MISSIONS - Editing & Scripting